Introduction
In an age where performance is king, it’s essential for developers to leverage technologies that optimize application speed and efficiency. When it comes to Node.js and Express, one of the most powerful tools at your disposal is Redis caching. This comprehensive guide will walk you through everything you need to know about integrating Redis into your Node.js and Express applications.
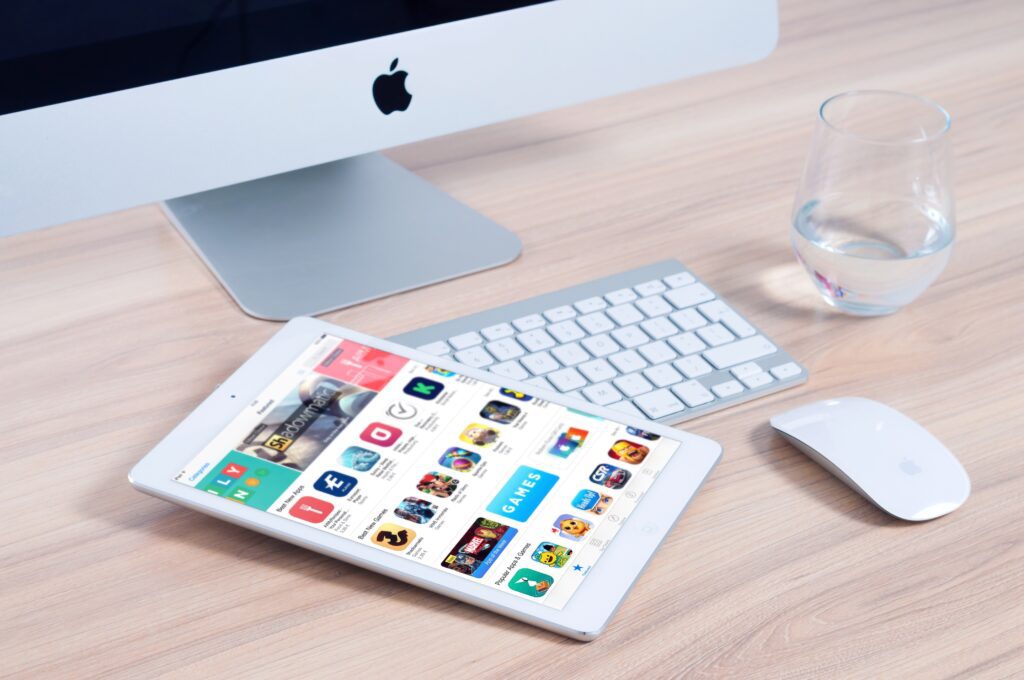
1. What is Redis?
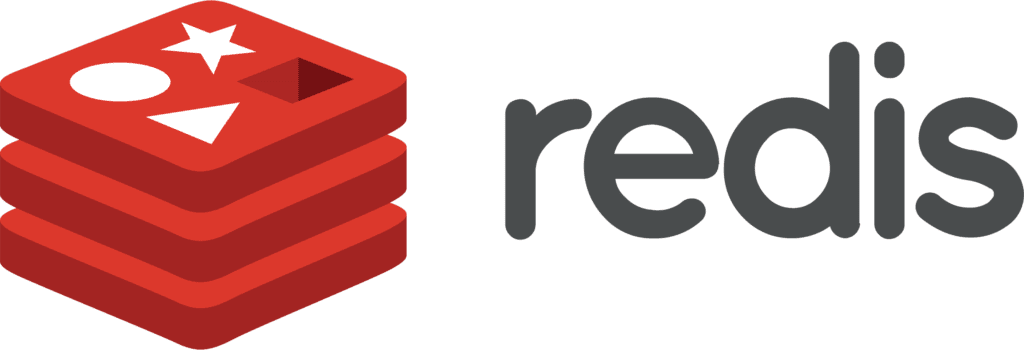
Redis, short for Remote Dictionary Server, is an open-source, in-memory data structure store that can be used as a database, cache, or message broker. It supports various data structures such as strings, hashes, lists, sets, and more. Being an in-memory store, Redis delivers exceptional performance by holding data in RAM, which is much faster than traditional disk-based databases.
Key Features of Redis:
- Exceptional performance
- Supports various data structures
- Persistence
- Replication
- Sharding
- Lua scripting
2. Why Use Redis with Node.js and Express?
Node.js, with its non-blocking architecture, is known for high performance. However, database queries, especially in large-scale applications, can become a bottleneck. This is where Redis comes in.
Integrating Redis into your Node.js and Express app can:
- Reduce latency: Redis’s in-memory storage drastically reduces the time taken to access data.
- Decrease load on your primary database: Caching frequent queries in Redis reduces the number of hits to your main database.
- Improve scalability: Faster data retrieval can significantly enhance your app’s ability to handle increased traffic.
3. Getting Started: Installing Redis
Before integrating Redis into your application, you need to install it on your machine. Visit the official Redis website for the latest version and installation instructions for your operating system.
For macOS:
brew install redis
For Ubuntu:
sudo apt-get update
sudo apt-get install redis-server
After installation, start the Redis server:
redis-server
4. Integrating Redis with Node.js and Express
To integrate Redis with your Node.js and Express app, you’ll need to use a Redis client. For this guide, we’ll use node-redis.
Install node-redis:
npm install redis
Now, let’s write some code:
const express = require('express');
const redis = require('redis');
const app = express();
const PORT = process.env.PORT || 3000;
// Create a Redis client
const client = redis.createClient();
// Handle Redis connection
client.on('connect', () => {
console.log('Connected to Redis...');
});
// Example route
app.get('/cache-test', (req, res) => {
// Key for Redis
const key = 'test-key';
// Check if data exists in cache
client.get(key, (err, result) => {
if (result) {
// Return cached data
return res.send(`Data from cache: ${result}`);
} else {
// Sample data
const data = "Data to be cached";
// Set data in cache
client.setex(key, 3600, data);
// Return data
return res.send(`Data from server: ${data}`);
}
});
});
// Start Express server
app.listen(PORT, () => {
console.log(`Server started on port ${PORT}`);
});
5. Cache Management Strategies
Cache management is critical for the optimal use of Redis. Here are some strategies:
- Cache Expiration: Setting an expiration time on your cache ensures that data doesn’t become stale.
- Least Recently Used (LRU) Caching: Redis can remove less recently used keys when a memory limit is reached.
- Namespacing: Group related data in the cache for easier management and invalidation.
6. Advanced Concepts
6.1. Redis Persistence
Redis provides two main mechanisms for disk persistence: RDB (snapshotting) and AOF (Append Only File). You can use these to persist data for long-term storage or recovery.
6.2. Redis Clustering
Redis supports sharding data across multiple Redis instances. With Redis Cluster, you can automatically split your dataset among several nodes.
Learn more about Redis Clustering
7. Best Practices
- Monitor Your Cache: Keep an eye on cache hit and miss ratios to ensure your cache is effectively improving performance.
- Connection Pooling: Reuse Redis connections rather than creating new ones for each request.
- Secure Your Redis Instance: Implement security best practices such as disabling unused commands, renaming critical commands, and configuring firewalls.
8. Common Pitfalls
- Overcaching: Storing too much data in the cache can lead to increased memory usage and potential bottlenecks.
- Cache Invalidation: Incorrectly invalidating cache can lead to serving stale data to clients.
FAQs
Can Redis be used as a primary database?
Yes, Redis has features like persistence and transactions which make it suitable as a primary database for certain use cases.
What is the difference between Redis and Memcached?
Redis supports a richer set of data types and has more advanced features like persistence and clustering, while Memcached is simpler and only supports string caching.
How do I secure my Redis instance?
Some steps include binding Redis to a secure private network, setting a strong password, and disabling or renaming dangerous commands.
Conclusion
Redis can be an extremely powerful tool when used in conjunction with Node.js and Express. It can significantly improve the performance and scalability of your application by reducing database load and latency. However, it is important to manage your cache effectively and monitor its usage to avoid common pitfalls.
Happy caching!