Introduction
Node.js and Express have revolutionized web development. With the ability to write server-side code in JavaScript, developers can create performant and scalable applications. However, with such power comes responsibility. It is easy to make mistakes that can lead to security vulnerabilities or performance issues. In this blog post, we’ll explore 10 common mistakes in Node.js and Express development and provide solutions for avoiding them.
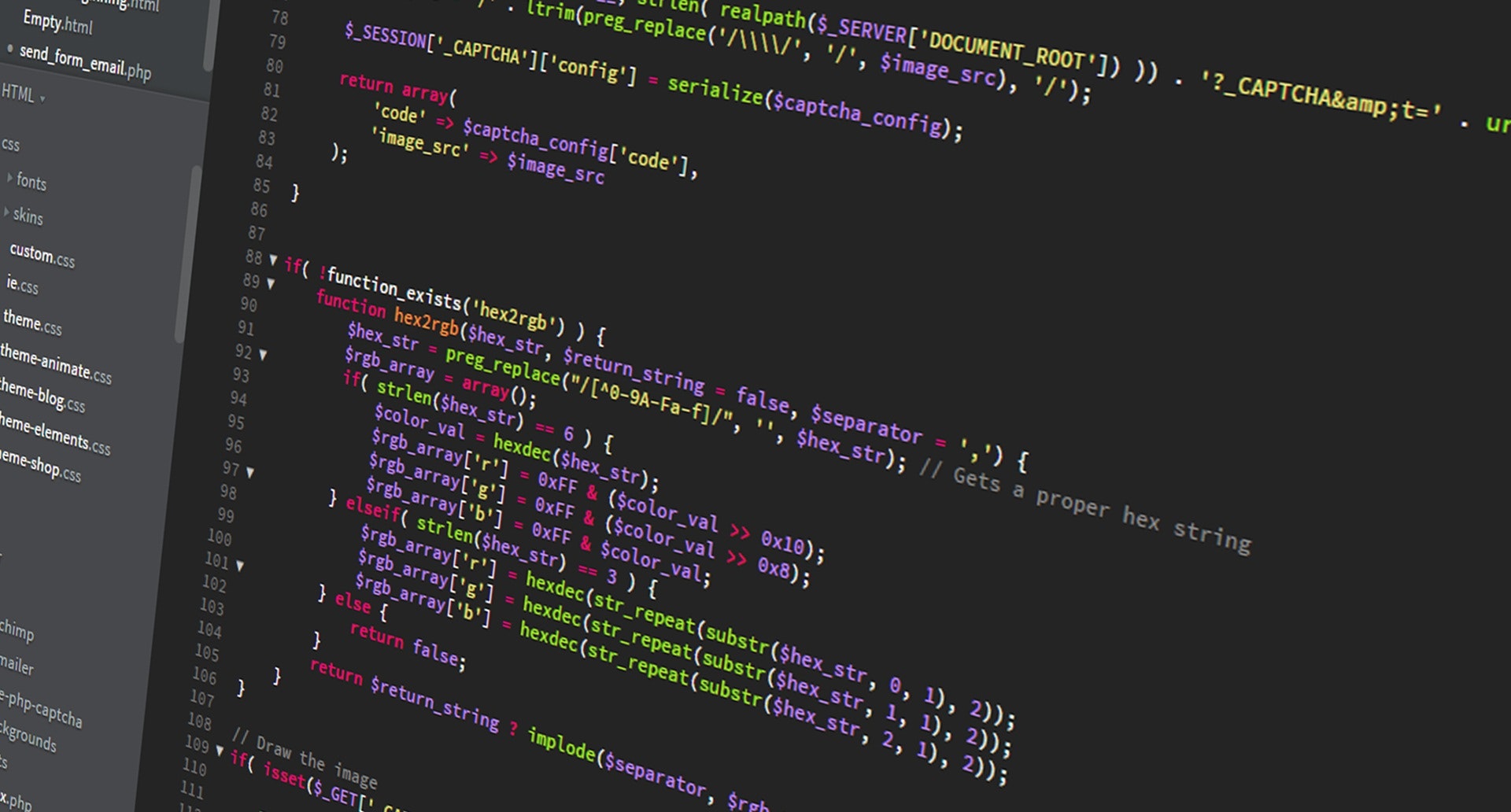
1. Neglecting Security
Security is paramount when it comes to web development. A common mistake is not sanitizing user input, which can lead to vulnerabilities such as SQL injection and Cross-Site Scripting (XSS).
Solution
Use libraries like helmet to secure your Express apps. Always validate and sanitize user input using libraries like validator or joi.
const express = require('express');
const helmet = require('helmet');
const app = express();
app.use(helmet());
2. Ignoring Error Handling
Failing to handle errors properly can result in an unstable application. Errors might occur during runtime that you didn’t anticipate during development.
Solution
Implement a global error handler in Express. Use the next
function to pass errors to this handler.
app.use((err, req, res, next) => {
console.error(err.stack);
res.status(500).send('Something broke!');
});
3. Misusing Middleware
Middleware functions are the backbone of Express applications. However, improper use can result in issues like a hung process or unintended side effects.
Solution
Place middleware functions in the right order and always call next()
to avoid hanging the response.
app.use((req, res, next) => {
// middleware logic here
next();
});
4. Blocking the Event Loop
Node.js is single-threaded, so blocking the event loop can make your application unresponsive.
Solution:
Avoid CPU-intensive tasks in the main thread. Use worker threads or offload the processing to a different service.
const { Worker, isMainThread } = require('worker_threads');
if (isMainThread) {
// This is the main thread.
const worker = new Worker(__filename);
} else {
// This is the worker thread.
// CPU-intensive task here
}
5. Not Optimizing Performance
Node.js is known for its performance, but not optimizing your code can slow down your application significantly.
Solution
Use caching, query optimization, and tools like PM2 for process management.
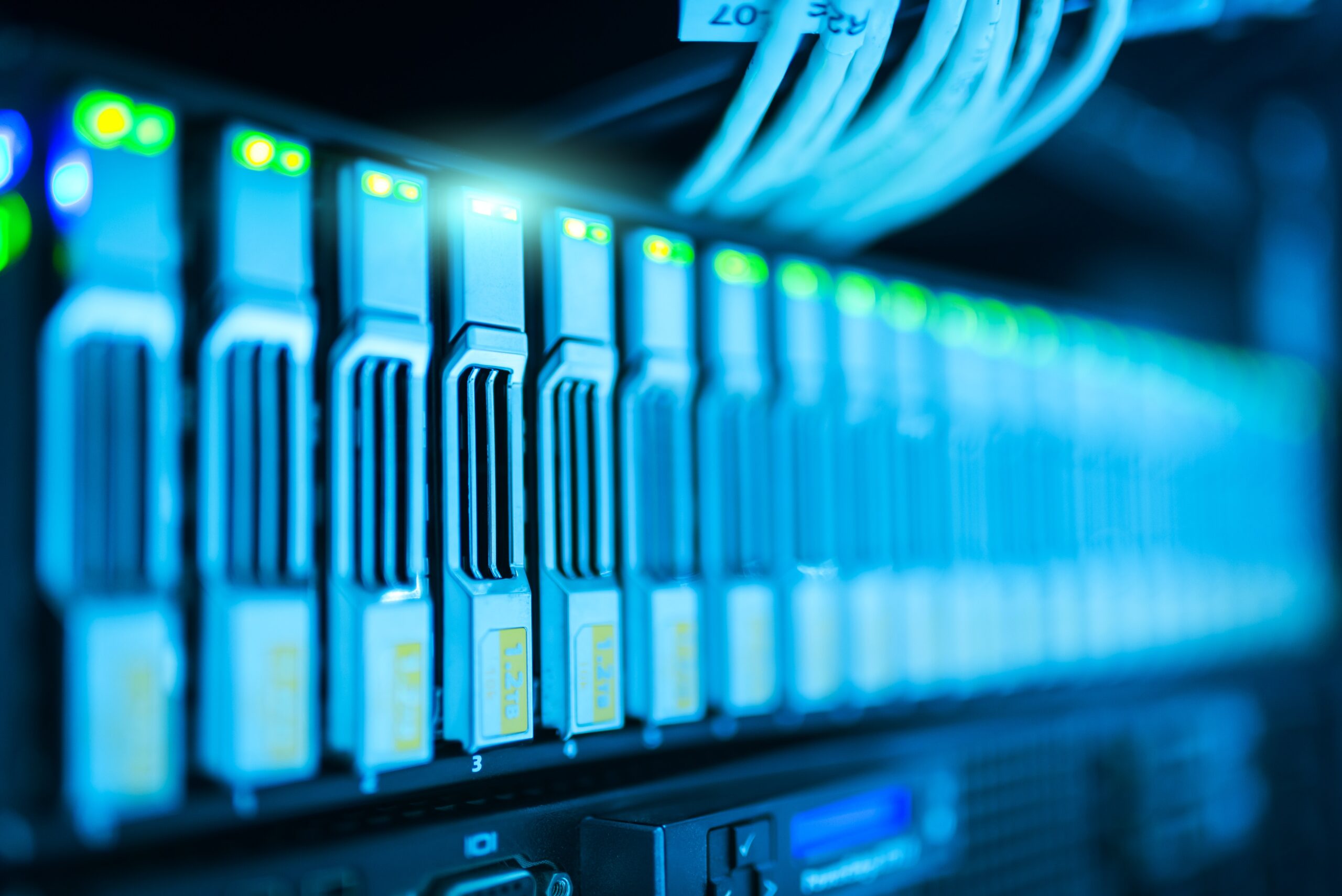
6. Callback Hell
Nested callbacks, known as “callback hell,” make your code unreadable and hard to maintain.
Solution
Use async/await
to write cleaner, more readable code.
async function fetchData() {
try {
const data = await someAsyncOperation();
// Process data
} catch (error) {
// Handle error
}
}
7. Not Using Environment Variables
Hardcoding configuration values and secrets in your code is a bad practice.
Solution
Use environment variables for configuration and secrets management. Use libraries like dotenv to load variables.
require('dotenv').config();
const secret = process.env.MY_SECRET;
8. Poor Logging Practices
Insufficient logging can make it difficult to diagnose issues in production.
Solution
Use logging libraries like winston or morgan and log relevant information.
const winston = require('winston');
const logger = winston.createLogger({
level: 'info',
format: winston.format.json(),
transports: [
new winston.transports.File({ filename: 'error.log', level: 'error' }),
new winston.transports.File({ filename: 'combined.log' })
]
});
9. Overlooking Code Quality
Maintaining code quality is essential for the long-term maintainability of your project.
Solution
Use linters like ESLint and follow a coding style guide. Keep your code DRY (Don’t Repeat Yourself) and write modular code.
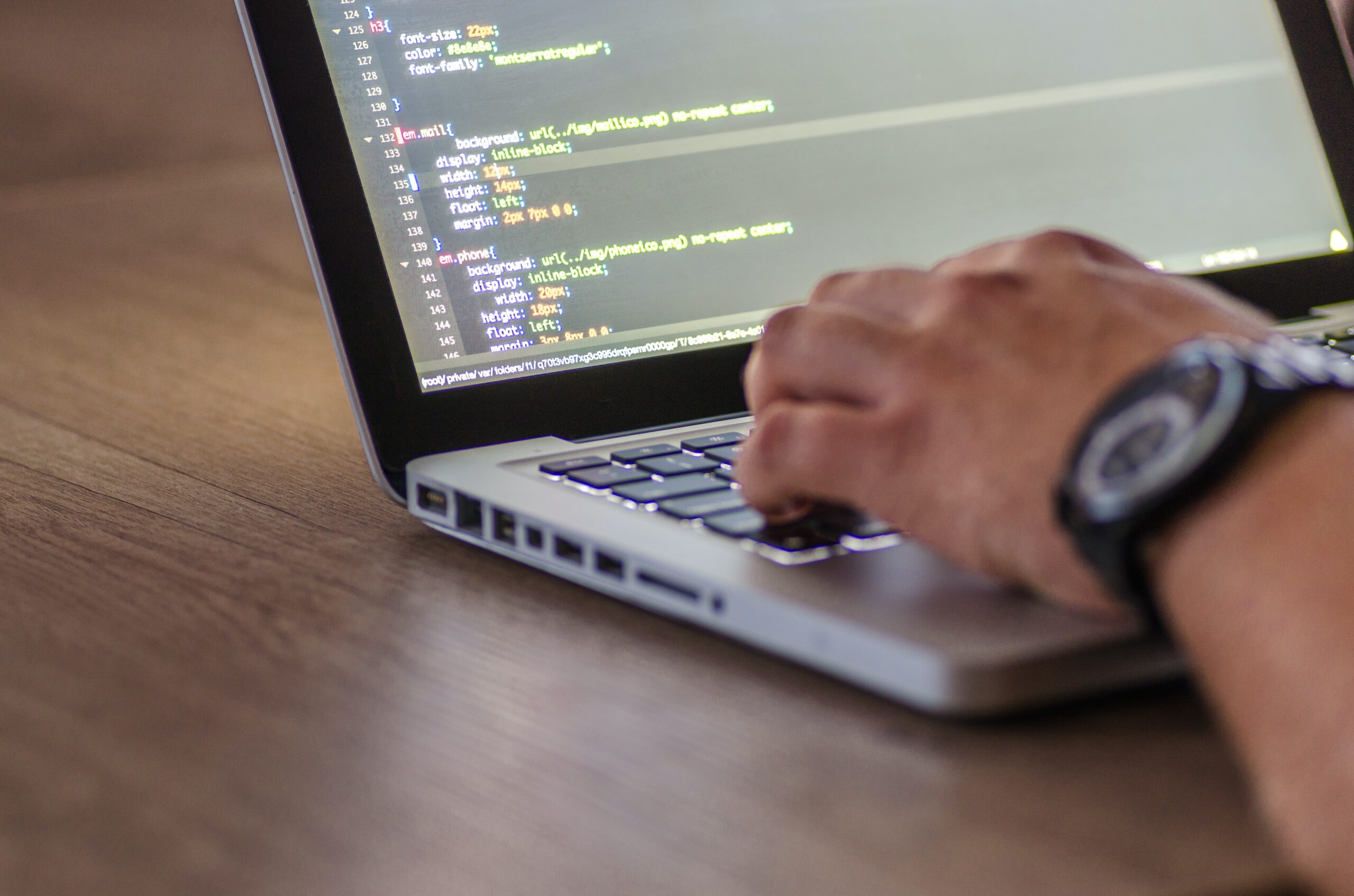
10. Not Keeping Dependencies Up to Date
Outdated dependencies can lead to security vulnerabilities and compatibility issues.
Solution
Regularly update your dependencies. Use npm outdated
to check for outdated packages and npm update
to update them.
npm outdated
npm update
Conclusion
Avoiding these common mistakes in Node.js and Express development can lead to more secure, maintainable, and efficient applications. By being mindful of security, error handling, performance optimization, and code quality, you can harness the full power of Node.js and Express.
FAQs
Is Node.js single-threaded?
Yes, Node.js is single-threaded, but it can still perform non-blocking I/O operations. For CPU-intensive tasks, you can use worker threads.
What is middleware in Express?
Middleware functions are functions that have access to the request and response objects, and the next middleware function in the application’s request-response cycle.
How do I handle errors in Express?
You can handle errors by using middleware functions with four arguments (err, req, res, next). Make sure to place this error-handling middleware after all other middleware functions and routes.
How can I optimize the performance of my Node.js app?
You can optimize performance by using caching, optimizing database queries, managing processes with PM2, and offloading CPU-intensive tasks.
What is callback hell and how can I avoid it?
Callback hell refers to heavily nested callbacks which make code hard to read and maintain. You can avoid it by using Promises or async/await.
Happy coding!
External resources: